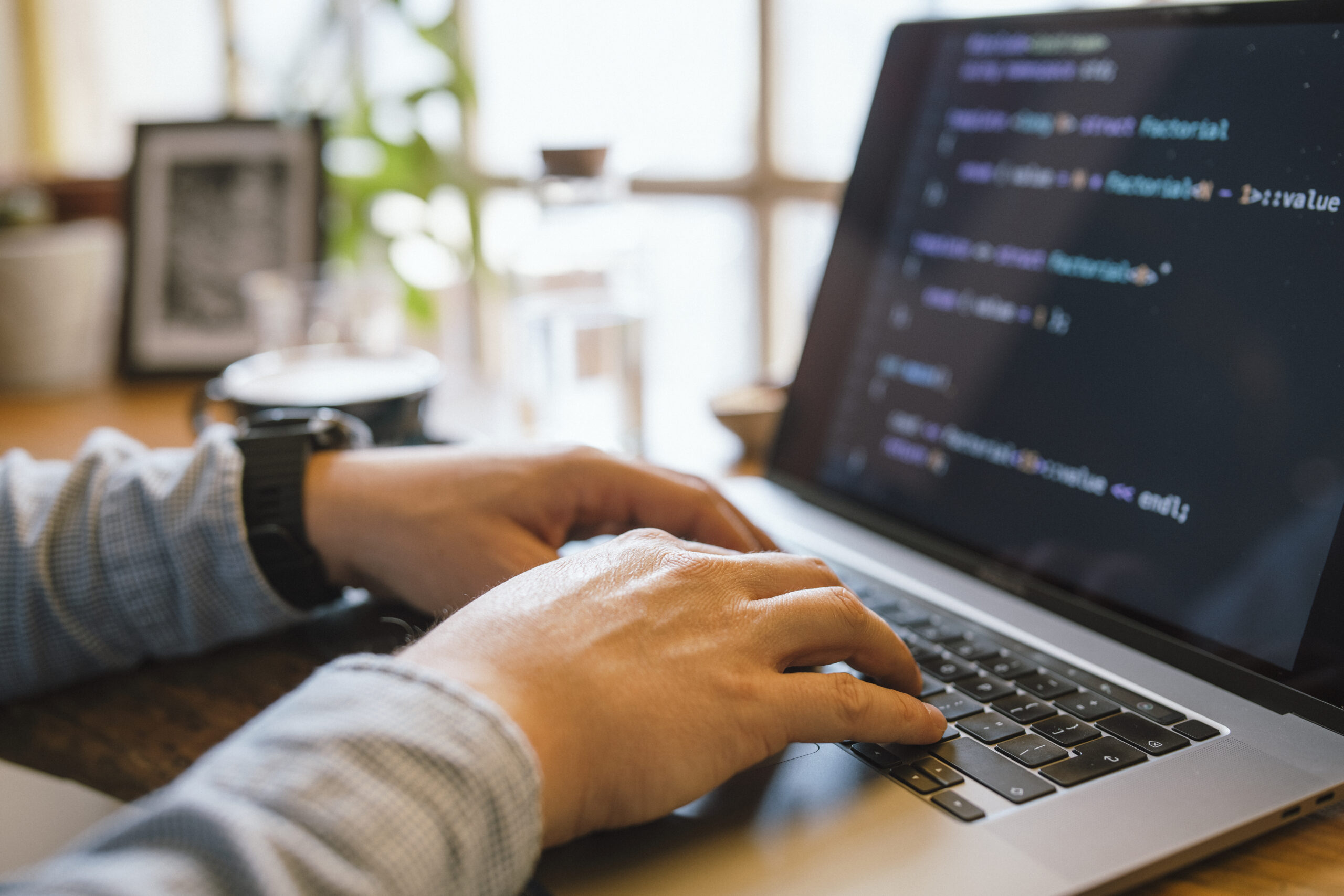
Debugging is One of the more critical — however typically forgotten — competencies in a developer’s toolkit. It isn't nearly repairing broken code; it’s about comprehension how and why things go wrong, and Studying to Assume methodically to unravel challenges competently. Regardless of whether you're a newbie or perhaps a seasoned developer, sharpening your debugging expertise can conserve hours of stress and substantially increase your productiveness. Allow me to share many approaches to help you builders amount up their debugging activity by me, Gustavo Woltmann.
Learn Your Instruments
Among the list of quickest strategies developers can elevate their debugging capabilities is by mastering the tools they use every single day. Though crafting code is one particular Component of growth, being aware of tips on how to communicate with it efficiently through execution is equally essential. Contemporary development environments come equipped with highly effective debugging abilities — but a lot of developers only scratch the surface area of what these resources can perform.
Take, for example, an Integrated Improvement Surroundings (IDE) like Visual Studio Code, IntelliJ, or Eclipse. These equipment help you set breakpoints, inspect the worth of variables at runtime, move by code line by line, and even modify code to the fly. When utilised properly, they Permit you to observe precisely how your code behaves in the course of execution, that is priceless for monitoring down elusive bugs.
Browser developer applications, for example Chrome DevTools, are indispensable for front-conclude developers. They assist you to inspect the DOM, monitor community requests, watch authentic-time effectiveness metrics, and debug JavaScript from the browser. Mastering the console, resources, and community tabs can convert discouraging UI problems into workable duties.
For backend or process-amount developers, tools like GDB (GNU Debugger), Valgrind, or LLDB give deep Regulate above functioning procedures and memory administration. Studying these instruments may have a steeper Understanding curve but pays off when debugging performance issues, memory leaks, or segmentation faults.
Outside of your IDE or debugger, grow to be snug with Edition Management systems like Git to comprehend code background, come across the exact instant bugs had been released, and isolate problematic adjustments.
In the end, mastering your instruments means heading outside of default configurations and shortcuts — it’s about creating an intimate knowledge of your growth ecosystem to ensure that when challenges come up, you’re not shed at midnight. The better you already know your applications, the greater time you can spend solving the actual difficulty as opposed to fumbling by way of the method.
Reproduce the challenge
One of the more crucial — and sometimes neglected — methods in powerful debugging is reproducing the trouble. Just before jumping into the code or creating guesses, developers need to produce a dependable ecosystem or state of affairs where by the bug reliably appears. Without having reproducibility, repairing a bug turns into a sport of chance, normally bringing about squandered time and fragile code improvements.
Step one in reproducing an issue is gathering just as much context as is possible. Question issues like: What actions led to The difficulty? Which natural environment was it in — growth, staging, or manufacturing? Are there any logs, screenshots, or mistake messages? The greater element you might have, the simpler it results in being to isolate the precise circumstances under which the bug occurs.
As you’ve gathered adequate information, seek to recreate the challenge in your local environment. This might suggest inputting exactly the same facts, simulating related person interactions, or mimicking program states. If the issue seems intermittently, think about producing automated exams that replicate the sting circumstances or state transitions associated. These tests not merely assistance expose the trouble but will also avoid regressions Down the road.
Sometimes, The problem may very well be atmosphere-distinct — it'd occur only on specified operating techniques, browsers, or less than specific configurations. Employing applications like virtual machines, containerization (e.g., Docker), or cross-browser screening platforms can be instrumental in replicating this kind of bugs.
Reproducing the challenge isn’t merely a move — it’s a mindset. It needs endurance, observation, along with a methodical technique. But when you finally can continuously recreate the bug, you're presently halfway to repairing it. That has a reproducible situation, You should utilize your debugging applications more successfully, check prospective fixes securely, and connect extra Evidently with your workforce or users. It turns an summary criticism right into a concrete challenge — Which’s where builders prosper.
Examine and Fully grasp the Mistake Messages
Error messages in many cases are the most beneficial clues a developer has when a little something goes Erroneous. In lieu of seeing them as annoying interruptions, builders really should discover to deal with error messages as direct communications from the method. They usually let you know what precisely happened, exactly where it happened, and occasionally even why it occurred — if you know how to interpret them.
Begin by looking at the concept very carefully and in complete. Many builders, particularly when under time stress, look at the initial line and immediately start building assumptions. But deeper within the mistake stack or logs may perhaps lie the legitimate root induce. Don’t just duplicate and paste error messages into search engines like yahoo — go through and understand them initial.
Split the mistake down into pieces. Can it be a syntax error, a runtime exception, or even a logic error? Will it place to a selected file and line number? What module or purpose brought on it? These issues can guideline your investigation and place you toward the accountable code.
It’s also useful to know the terminology of the programming language or framework you’re employing. Mistake messages in languages like Python, JavaScript, or Java usually follow predictable designs, and Understanding to recognize these can considerably quicken your debugging course of action.
Some errors are obscure or generic, As well as in those situations, it’s essential to look at the context wherein the error occurred. Test related log entries, input values, and up to date variations from the codebase.
Don’t overlook compiler or linter warnings both. These generally precede larger concerns and provide hints about opportunity bugs.
Ultimately, mistake messages are usually not your enemies—they’re your guides. Studying to interpret them accurately turns chaos into clarity, serving to you pinpoint issues quicker, lessen debugging time, and turn into a more productive and self-confident developer.
Use Logging Sensibly
Logging is one of the most potent resources in the developer’s debugging toolkit. When applied proficiently, it provides actual-time insights into how an application behaves, aiding you realize what’s taking place beneath the hood while not having to pause execution or phase throughout the code line by line.
A very good logging system starts with knowing what to log and at what level. Common logging levels include DEBUG, Facts, Alert, Mistake, and Lethal. Use DEBUG for in-depth diagnostic information and facts during development, Facts for normal events (like thriving begin-ups), Alert for probable troubles that don’t split the application, ERROR for precise challenges, and Deadly if the program can’t carry on.
Avoid flooding your logs with too much or irrelevant knowledge. A lot of logging can obscure essential messages and decelerate your technique. Center on crucial occasions, point out adjustments, input/output values, and significant selection details as part of your code.
Structure your log messages clearly and continuously. Incorporate context, including timestamps, request IDs, and function names, so it’s simpler to trace troubles in distributed techniques or multi-threaded environments. Structured logging (e.g., JSON logs) can make it even simpler to parse and filter logs programmatically.
In the course of debugging, logs Permit you to observe how variables evolve, what circumstances are achieved, and what branches of logic are executed—all with no halting the program. They’re especially worthwhile in production environments wherever stepping via code isn’t probable.
Furthermore, use logging frameworks and applications (like Log4j, Winston, or Python’s logging module) that help log rotation, filtering, and integration with checking dashboards.
In the end, wise logging is about stability and clarity. Which has a nicely-considered-out logging approach, you may lessen the time it will take to identify problems, achieve further visibility into your applications, and Enhance the Over-all maintainability and trustworthiness of one's code.
Consider Like a Detective
Debugging is not only a technological job—it's a sort of investigation. To correctly determine and resolve bugs, builders will have to method the process similar to a detective solving a mystery. This attitude will help stop working elaborate issues into manageable elements and stick to clues logically to uncover the foundation cause.
Begin by gathering evidence. Consider the signs of the challenge: mistake messages, incorrect output, or effectiveness challenges. Much like a detective surveys a crime scene, collect as much related info as you'll be able to without having leaping to conclusions. Use logs, take a look at conditions, and person reports to piece together a clear picture of what’s happening.
Subsequent, kind hypotheses. Question by yourself: What may be causing this behavior? Have any changes lately been created to your codebase? Has this difficulty occurred ahead of beneath very similar conditions? The purpose is always to narrow down possibilities and identify potential culprits.
Then, test your theories systematically. Try to recreate the condition in a very managed ecosystem. For those who suspect a certain purpose or element, isolate it and verify if the issue persists. Similar to a detective conducting interviews, talk to your code issues and Allow the final results direct you closer to the reality.
Shell out close attention to small aspects. Bugs often cover within the minimum envisioned spots—like a missing semicolon, an off-by-just one mistake, or perhaps a race affliction. Be complete and affected person, resisting the urge to patch The difficulty without having totally understanding it. Short term fixes may conceal the actual issue, just for it to resurface later on.
Lastly, hold notes on what you experimented with and learned. Equally as detectives log their investigations, documenting your debugging system can preserve time for upcoming problems and assistance Other people fully check here grasp your reasoning.
By considering just like a detective, builders can sharpen their analytical skills, strategy challenges methodically, and turn out to be simpler at uncovering concealed challenges in complex units.
Write Exams
Producing checks is among the most effective approaches to increase your debugging competencies and General advancement performance. Tests not just enable capture bugs early but will also function a safety Internet that provides you assurance when making modifications on your codebase. A perfectly-tested application is easier to debug since it lets you pinpoint particularly where and when an issue happens.
Begin with unit checks, which deal with individual functions or modules. These little, isolated tests can rapidly reveal no matter whether a certain piece of logic is Doing work as anticipated. Any time a exam fails, you immediately know where to appear, considerably decreasing the time used debugging. Device checks are Primarily useful for catching regression bugs—troubles that reappear right after Formerly getting set.
Next, combine integration assessments and conclusion-to-conclusion assessments into your workflow. These aid make certain that several areas of your application work alongside one another efficiently. They’re notably beneficial for catching bugs that happen in elaborate devices with a number of components or products and services interacting. If a little something breaks, your exams can tell you which Component of the pipeline failed and less than what situations.
Writing assessments also forces you to Assume critically regarding your code. To test a feature correctly, you need to be aware of its inputs, anticipated outputs, and edge circumstances. This level of comprehension naturally sales opportunities to better code framework and much less bugs.
When debugging a problem, producing a failing examination that reproduces the bug can be a strong starting point. Once the examination fails consistently, it is possible to give attention to correcting the bug and view your take a look at pass when The problem is resolved. This technique makes certain that precisely the same bug doesn’t return Down the road.
In brief, producing checks turns debugging from a aggravating guessing video game right into a structured and predictable method—serving to you capture far more bugs, a lot quicker and much more reliably.
Just take Breaks
When debugging a tough issue, it’s straightforward to become immersed in the situation—staring at your screen for hrs, striving Alternative following Alternative. But one of the most underrated debugging resources is just stepping away. Using breaks will help you reset your head, lower frustration, and often see the issue from a new perspective.
When you're also near to the code for also prolonged, cognitive tiredness sets in. You could possibly start out overlooking evident errors or misreading code that you simply wrote just hours earlier. Within this state, your Mind results in being much less effective at issue-solving. A brief wander, a espresso break, or perhaps switching to a different task for ten–quarter-hour can refresh your target. Several developers report obtaining the basis of an issue after they've taken the perfect time to disconnect, permitting their subconscious operate inside the background.
Breaks also help reduce burnout, In particular through more time debugging sessions. Sitting down before a display screen, mentally stuck, is don't just unproductive and also draining. Stepping away allows you to return with renewed Electricity plus a clearer state of mind. You might quickly recognize a lacking semicolon, a logic flaw, or possibly a misplaced variable that eluded you prior to.
If you’re trapped, a superb rule of thumb is usually to set a timer—debug actively for forty five–60 minutes, then have a 5–ten minute crack. Use that time to maneuver about, extend, or do some thing unrelated to code. It may sense counterintuitive, Specifically less than tight deadlines, but it really truly causes more quickly and more practical debugging Over time.
To put it briefly, using breaks is not really a sign of weak point—it’s a sensible method. It provides your Mind space to breathe, enhances your perspective, and will help you steer clear of the tunnel vision That usually blocks your development. Debugging is really a mental puzzle, and relaxation is an element of solving it.
Study From Every Bug
Every single bug you experience is much more than simply a temporary setback—It truly is a possibility to grow being a developer. Whether it’s a syntax mistake, a logic flaw, or perhaps a deep architectural situation, each can train you one thing worthwhile if you make an effort to replicate and evaluate what went wrong.
Begin by asking on your own a few critical thoughts once the bug is resolved: What triggered it? Why did it go unnoticed? Could it are already caught before with better methods like unit tests, code reviews, or logging? The answers usually reveal blind spots inside your workflow or comprehension and assist you Construct more powerful coding practices transferring ahead.
Documenting bugs can even be an outstanding pattern. Continue to keep a developer journal or manage a log in which you note down bugs you’ve encountered, how you solved them, and what you realized. With time, you’ll start to see styles—recurring challenges or prevalent problems—you can proactively prevent.
In staff environments, sharing Whatever you've uncovered from a bug together with your peers is usually Primarily potent. Whether or not it’s through a Slack information, a short create-up, or A fast expertise-sharing session, supporting Other individuals stay away from the same difficulty boosts workforce effectiveness and cultivates a more robust Understanding culture.
Additional importantly, viewing bugs as lessons shifts your frame of mind from stress to curiosity. As opposed to dreading bugs, you’ll start appreciating them as necessary elements of your enhancement journey. All things considered, a few of the greatest builders will not be those who publish perfect code, but individuals that constantly master from their blunders.
Eventually, Every single bug you repair provides a fresh layer for your ability set. So upcoming time you squash a bug, take a minute to replicate—you’ll come away a smarter, extra capable developer as a consequence of it.
Conclusion
Strengthening your debugging competencies normally takes time, practice, and persistence — though the payoff is huge. It would make you a more economical, confident, and capable developer. The subsequent time you might be knee-deep inside a mysterious bug, don't forget: debugging isn’t a chore — it’s a possibility to become far better at That which you do.